/Bathroom/TowelRail2.foocad
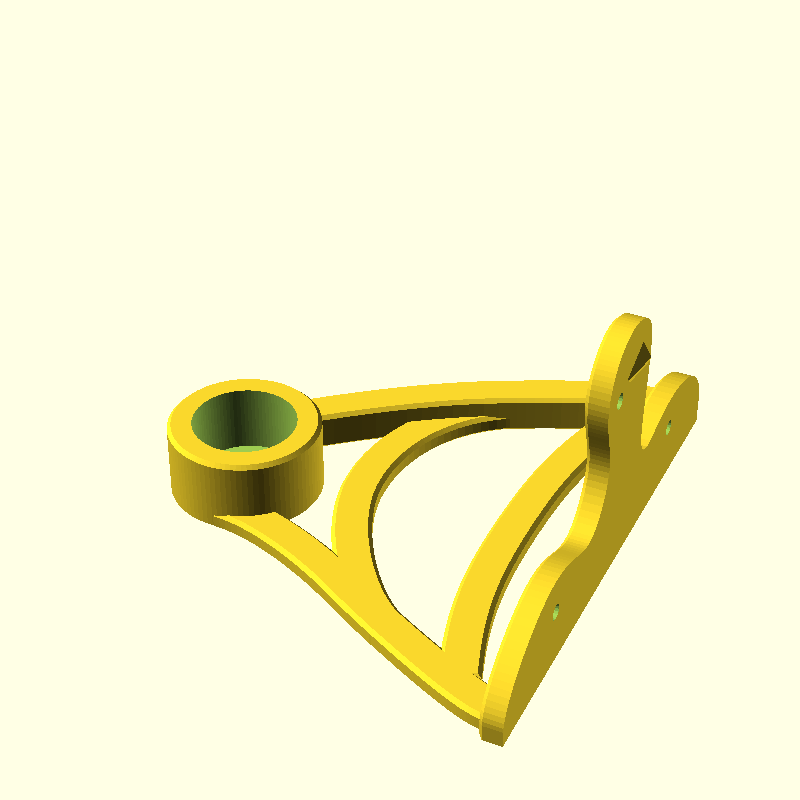
A towel rail which screws to a wall. The rail itself is wood.
See TowelRail.foocad for a version which hooks over a shower screen.
Usage
Use rounded headed screws.
import static uk.co.nickthecoder.foocad.chamferedextrude.v1.ChamferedExtrude.* class TowelRail2 : Model { var thickness = 10 var depth = 5 var capSize = 20 var chamfer = 1 var poleD = 26.5 fun test( shape : Shape2d ) : Shape3d { return shape.firstPath.thickness( thickness ).extrude(0.4) } fun thicken( shape : Shape2d ) = thicken( shape, depth ) fun thicken( shape : Shape2d, depth : double ) : Shape3d { return shape.firstPath.thickness( thickness ).chamferedExtrude( depth, chamfer ) } @Piece fun mirror() = build().mirrorX() override fun build() : Shape3d { val shapes = SVGParser().parseFile( "towelRail2.svg" ).shapes val a = thicken( shapes["a"], depth*2 ) val b = thicken( shapes["b"] ) val c = thicken( shapes["c"] ) val d = thicken( shapes["d"] ) val cap = shapes["cap"].chamferedExtrude( capSize, chamfer ) val middle = thicken( shapes["middle"], depth*2 ).rotateY(30).bottomTo(cap.top - depth*2 - 4) - Cube( 1000 ).center().rightTo(a.left) val pos = shapes["cap"].middle val recess = Circle.hole(poleD/2).translate( pos ) .extrude(capSize*2) .translateZ(depth/2) val end = Circle( thickness/2 ).translate( shapes["a"].firstPath.reverse().points[0] ).chamferedExtrude( depth*2, chamfer ) val most = (a+b+c+d + middle + cap - recess).toOriginY() val screwHoles = Cylinder(20,2).center().rotateY(90).translate(0, 30, 15).translate(0,78,0).also() val extraHole = Cylinder(20,2).center().rotateY(90).translate( 0, 64, 50 ) val mount = ( shapes["mount"].chamferedExtrude( 6, 0, 1 ) .rotateY(90).toOrigin() - screwHoles ).translateY( most.size.y - shapes["mount"].size.y + 2 ) - extraHole val all = most + mount return all.mirrorX() } }