/Bedroom/PhonePouch.foocad
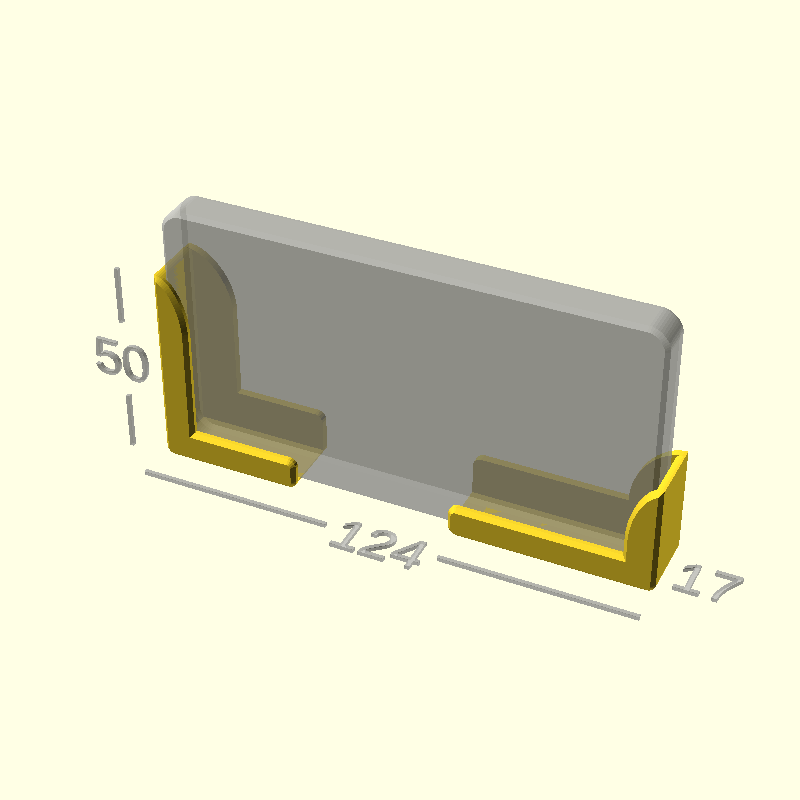
I want a place to put my phone overnight, while charging. My solution is a pouch, which is fixed to my bedside table. It consists of two small corner pieces, stuck to the table the right distance apart for the length of my phone.
The design is deliberately asymmetric, for acces to the USB port.
Notes
To hold the phone vertically, print 2 of the smaller corners. Miror images of each other.
import static uk.co.nickthecoder.foocad.chamferedextrude.v1.ChamferedExtrude.* class PhonePouch : AbstractModel() { @Custom( about="Thickness of your phone, with added slack" ) var phoneT = 13 @Custom( about="The size of the left half" ) var sizeL = Vector2( 35, 50 ) @Custom( about="THe size of the right half" ) var sizeR = Vector2( 50, 28 ) @Custom( about="The width of the horizontal and vertical parts") var frontWidth = 8 @Custom var mirror = false var backWidth = 12 var thickness = 2.0 var backT = 2.0 fun profile(size : Vector2, width : double) : Shape2d { val largeR = 15 val medR = 2 return PolygonBuilder().apply { moveTo(0, 0 ) lineBy( width, 0 ) lineBy( 0, -size.y+width ) lineBy( size.x- width, 0 ) lineBy( 0, -width ) lineBy( -size.x , 0 ) }.build().toOrigin() .roundCorner(4, largeR) .roundCorner(2, medR) .roundCorner(1, medR) .roundCorner(0, medR) } fun half( size : Vector2 ) : Shape3d { val backProfile = profile(size, backWidth) val back = backProfile.extrude( backT ) val frontProfile = profile(size, frontWidth) val middleP = frontProfile - frontProfile.offset( - thickness ) - Square( frontProfile.size ).translate(thickness, thickness ) val middle = middleP.extrude( phoneT ) .bottomTo(back.top) val front = frontProfile.chamferedExtrude( thickness, 0, 1 ) .bottomTo(middle.top) return back + middle + front } @Piece fun leftHalf() = half( sizeL ).rotateX(90) @Piece fun rightHalf() = half( sizeR ).rotateX(90).mirrorX() override fun build() : Shape3d { val phone = Square( 120, 65 ).roundAllCorners( 5 ) .chamferedExtrude(10,1) .rotateX(90) .centerXY() .previewOnly() val leftHalf : Shape3d = leftHalf().centerY() .leftTo( phone.left - thickness ) val rightHalf : Shape3d = rightHalf().centerY() .rightTo( phone.right + thickness ) return leftHalf + phone + rightHalf } }