/Boxes/CR2032Box.foocad
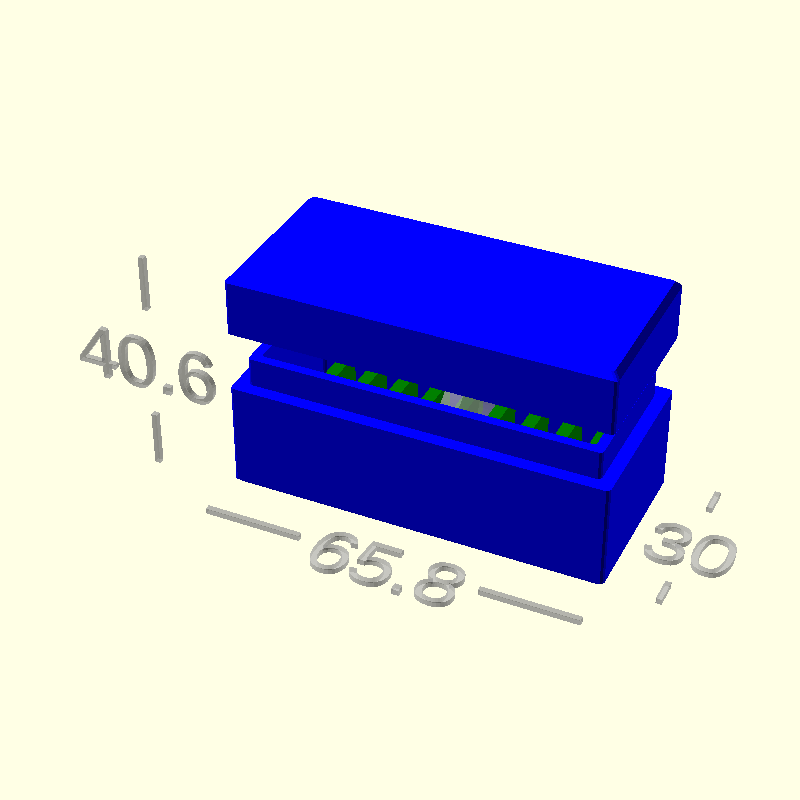
A small box to hold CR2032 batteries.
import static uk.co.nickthecoder.foocad.layout.v1.Layout2d.* import static uk.co.nickthecoder.foocad.layout.v1.Layout3d.* include ThickWalledBox.foocad class MyBox : ThickWalledBox() { override fun profile() : Shape2d = super.profile().roundAllCorners(0.5) } class CR2032Box : Model { var batteries = 10 var batteryT = 3.8 // Extra space than 3.2 for a CR2032 var batteryD = 22 var gap = 2.2 fun box() = MyBox().apply { width = (batteryT+gap) * batteries - gap depth = batteryD bottomHeight = batteryD*0.7 topHeight = batteryD*0.3 chamfer = 1 } @Piece fun bottom() : Shape3d { val slot = Cube(gap,batteryD+1, batteryD*0.5).centerXY() val slots = slot.tileX( batteries-1, batteryT ) .centerX() .bottomTo(2) .color("Green") val box : MyBox = box() val bottom : Shape3d = box.bottom() return slots + bottom } @Piece fun top() = box().top() override fun build() : Shape3d { val battery = Cylinder( 3.2, 20/2 ).rotateY(90) .bottomTo( box().thickness ) .leftTo(gap/2) .previewOnly() return top().rotateY(180).bottomTo( 30 ) + bottom()+ battery } }