/Boxes/ComponentDrawer.foocad
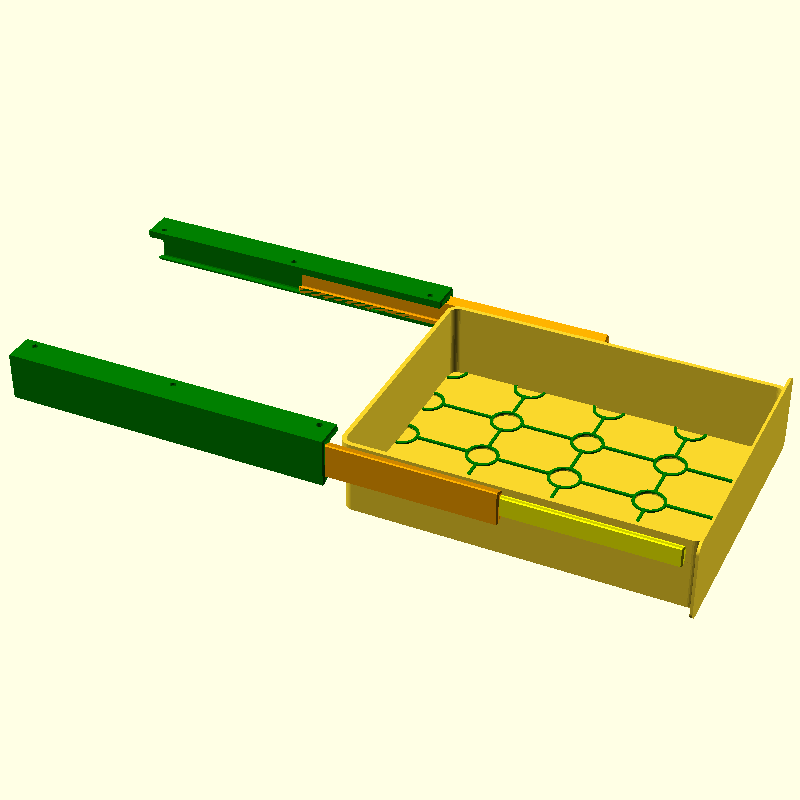
Creates drawers which can be attached to the bottom of a shelf / cupboard. The drawers have a grid for "inserts" from ComponentOrganiset.foocad. The drawers open/close with the help of DrawerSlide.foocad.
Glue a pair of "inner" slides to the drawer. When the glue is dry, attach the middle and outer and screw the outer to the shelf/cupboard. Ensure that the slides are parallel.
import static uk.co.nickthecoder.foocad.layout.v1.Layout2d.* import static uk.co.nickthecoder.foocad.layout.v1.Layout3d.* include ComponentOrganiser.foocad include DrawerSlide.foocad class ComponentDrawer : Model { @Custom var size = Vector2( 4, 4 ) val baseChamfer = 1 val baseThickness = 1.4 val height = 52 val thickness = 1.2 val ridge = Vector2(1.8, 16) @Piece fun drawer() : Shape3d { //println( "Size : ${ComponentOrganiser().insert(1,1).size}" ) val co = componentOrganiser() val inserts : Shape3d = inserts(co) val drawerBottom = co.drawerBottom().centerXY().bottomTo( baseThickness ).color("Green") val profile = Square( inserts.size.x, inserts.size.y ) .roundAllCorners(4) .center() val fatterBase = (profile.offset(1) - co.footwells()).extrude(2) val drawer = ExtrusionBuilder().apply { // Chamfered base crossSection( profile.offset( -baseChamfer + thickness + 1) ) forward( baseChamfer ) crossSection( baseChamfer ) // Main upright forward( height - baseChamfer - baseThickness - ridge.x - ridge.y) crossSection() crossSection() // Ridge forward( ridge.x ) crossSection( ridge.x ) forward( ridge.y ) crossSection() crossSection( -ridge.x - thickness ) // Back down forward( -height + baseChamfer + baseThickness ) crossSection() // Bottom. //forward( -baseChamfer ) //crossSection( - baseChamfer ) }.build() return drawer + drawerBottom + fatterBase } @Piece fun drawerFronted() : Shape3d { val plain = drawer() val left = plain.right - thickness - ridge.x val removeFront = Square( plain.size.z + 4, plain.size.y + 18 ) .extrude( 100 ) .rotateY(-90) .centerY() .leftTo( left ) val front = Square( plain.size.z + 4, plain.size.y + 18 ) .roundAllCorners( 6 ) .extrude( ridge.x ) .rotateY(-90) .centerY() .leftTo( left ) return plain - removeFront + front } @Piece fun inner() : Shape3d { return slide().inner() } @Piece fun middle() : Shape3d { return slide().middle() } @Piece fun outerB() : Shape3d { return slide().apply { tabCount = 4 }.outerB() } @Piece fun sliderSet() : Shape3d { val outers = outerB().leftTo(1).mirrorX().also() val middles = middle().leftTo( outers.right + 2 ).mirrorX().also() val inners = inner().leftTo( middles.right + 2 ).mirrorX().also() return outers + middles + inners } override fun build() : Shape3d { val plain = drawer() val drawer = drawerFronted() val slides = slide().build() .rotateZ(90).rotateX(-90) .frontTo( plain.front - 11 ) .rightTo( plain.right - 11 ) .translateZ( 40 ) .mirrorY().also() return drawer + slides } fun slide() = DrawerSlide().apply { length = 220 holeCount = 0 } fun componentOrganiser() = ComponentOrganiser().apply { drawerX = size.x drawerY = size.y } fun inserts( co : ComponentOrganiser ) : Shape3d { return co.insert(1,1) .tileX( size.x, co.insertGap ).tileY( size.y, co.insertGap ) .previewOnly() .centerXY() } }