/Boxes/StackingComponentBox.foocad
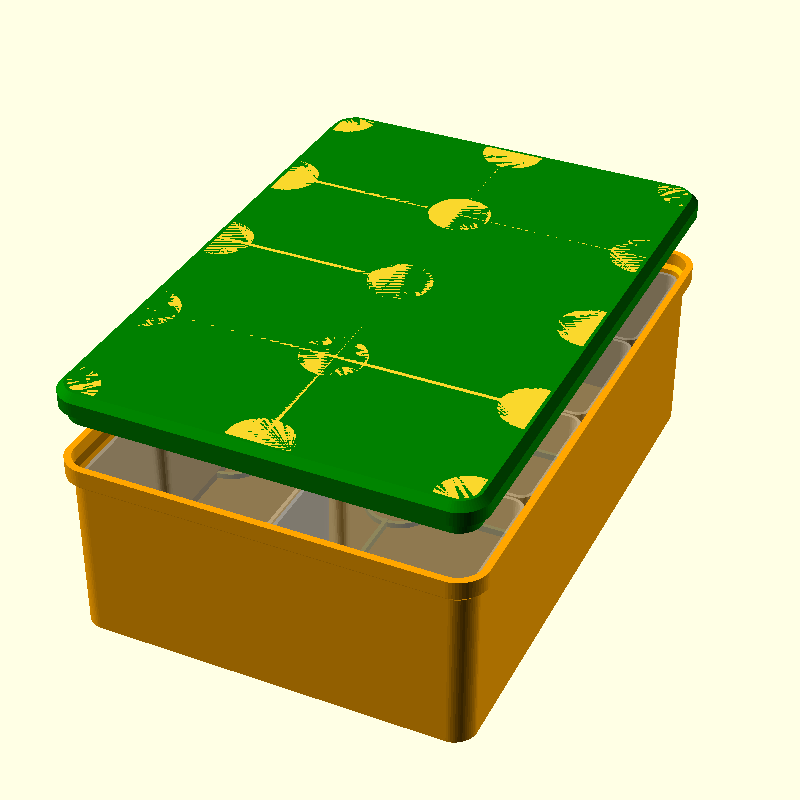
A stacking box, which will contain standard sized component organiser inserts from ComponentOrganiser.foocad.
Oops. I later created a near-duplicate of this model called ComponentOrganiserStacking.
Print Notes
main
Use many perimeters, which is better than it doing infill when it gets to the thick bit at the top.
lid
10% infill, and 2 perimeters is fine.
import static uk.co.nickthecoder.foocad.layout.v1.Layout2d.* import static uk.co.nickthecoder.foocad.layout.v1.Layout3d.* include StackingBox.foocad include ComponentOrganiser.foocad class StackingComponentBox : AbstractModel() { @Custom var size = Vector2( 2, 4 ) @Piece( printable = false ) override fun build() : Shape3d { val main : Shape3d = main() val lid : Shape3d = lid().mirrorZ() return main + lid.bottomTo( main.top + 10 ) } fun componentOrganiser() = ComponentOrganiser().apply { drawerX = size.x drawerY = size.y } fun inserts( co : ComponentOrganiser ) : Shape3d { return co.insert(1,1) .tileX( size.x, co.insertGap ).tileY( size.y, co.insertGap ) .previewOnly() .centerXY() } @Piece fun main() : Shape3d { //println( "Size : ${ComponentOrganiser().insert(1,1).size}" ) val co = componentOrganiser() val inserts : Shape3d = inserts(co) val sb = StackingBox().apply { boxWidth = inserts.size.x // 54*size.x boxDepth = inserts.size.y //39*size.y boxHeight = 49 } co.drawerThickness = sb.baseThickness val drawerBottom = co.drawerBottom().centerXY() val box = sb.main() return box + inserts.bottomTo( drawerBottom.top ) + drawerBottom } @Piece fun lid() : Shape3d { val co = componentOrganiser() val inserts : Shape3d = inserts(co) val sb = StackingBox().apply { boxWidth = inserts.size.x // 54*size.x boxDepth = inserts.size.y //39*size.y boxHeight = 49 } val slatT = sb.thickness val lid = sb.lid() val ys = Cube( inserts.size.x + 2, slatT, lid.size.z ) .repeatY( size.y-1, inserts.size.y/size.y ) .centerXY() val xs = Cube( slatT, inserts.size.y+2, lid.size.z ) .repeatX( size.x-1, inserts.size.x/size.x ) .centerXY() val cornerR = 9 val corners = Circle( cornerR ) .repeatX( size.x+1, inserts.size.x/size.x ) .repeatY( size.y+1, inserts.size.y/size.y ) .center() val castle = Circle( cornerR ) / (Square(10).roundAllCorners(co.insertRadius+1).translate(3,3) .mirrorX().also().mirrorY().also()) val castles = castle .repeatX( size.x+1, inserts.size.x/size.x ) .repeatY( size.y+1, inserts.size.y/size.y ) .center() val within = Square( inserts.size.x+2, inserts.size.y+2 ) .roundAllCorners(sb.round - sb.thickness) .center() val details = (corners / within).extrude( lid.size.z ) + (castles / within).extrude( lid.size.z + 3 ) return lid + xs + ys + details //- //details.mirrorZ().topTo( 0.8 ) //- //xs.topTo( 0.8 ) - ys.topTo( 0.8 ) } }