/Boxes/WoodenComponentCabinet.foocad
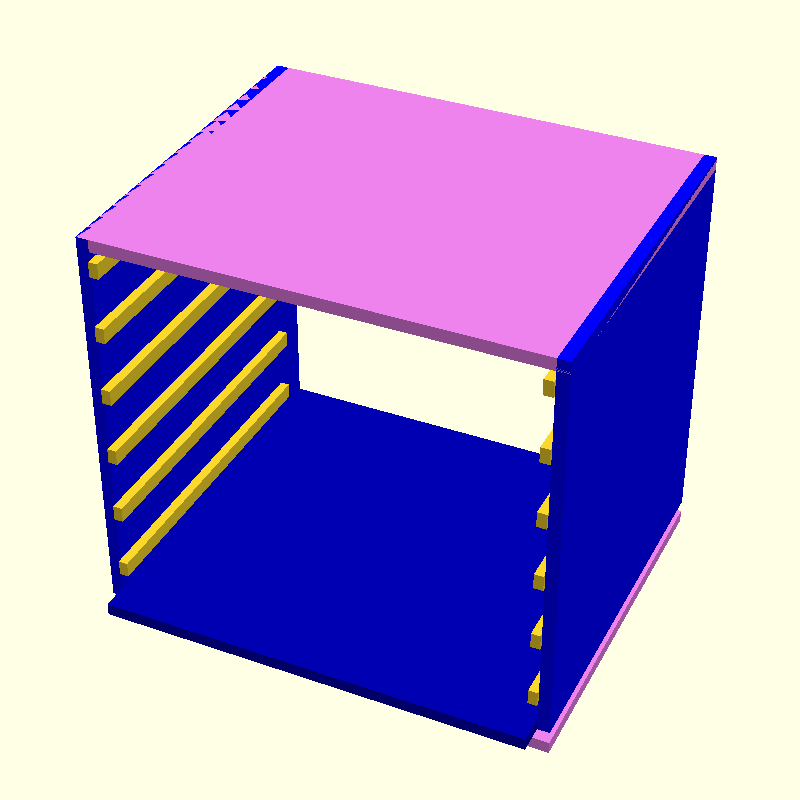
A simple box, with wooden runners to house my B&Q component organiser boxes. https://www.diy.com/departments/20-compartment-compartment-organiser-case/1345784_BQ.prd
NOTE. I will make one of the boxes fixed in place, with full extension drawer runners, so that I can use it as a shelf (as somewhere to place each box when it is removed from the cabinet).
import static uk.co.nickthecoder.foocad.along.v2.Along.* import static uk.co.nickthecoder.foocad.layout.v1.Layout2d.* import static uk.co.nickthecoder.foocad.layout.v1.Layout3d.* class WoodenComponentCabinet : Model { // Thickness of the plywood var thickness = 12 // Should include a little wiggle room! This is the internal width of the box. var boxWidth = 420 // Must fit 350mm drawer runners! var boxDepth = 350 // Should include extra wiggle room. var boxHeight = 65 // If there needs to be extra space between boxes, but not at the bottom and // top of the cabinter var gap = 0 var drawerCount = 6 // Dimensions of the wood for the wooden slides. // The width is quite critical, but the height is fairly arbitrary. var slideW = 9 var slideH = 15 // How far into the cabinet the slides start. My boxes have a natural "stop" // near the front, so the slides cannot start at the front of the cabinet. var slideShort = 25 val ply = SheetLumber( "ply", thickness ) val slideL = Lumber( "slide", slideW, slideH ) fun cabinet() : Shape3d { val width = boxWidth + thickness * 2 val depth = boxDepth + thickness val height = drawerCount * ( boxHeight + gap ) - gap + thickness * 2 val topBottom = ply.cut( width, depth ) .color("Violet").label("bottom") .alongY2().centerXY() .translateZ(height-thickness).also() val sides = ply.cut( height - thickness*2, depth ) .color("Blue").label("side") .alongY() .translateZ(thickness) .centerY().leftTo(topBottom.left).mirrorX().also() val back = ply.cut( height - thickness*2, width - thickness*2) .color("Blue").darker().label("back") .alongX().centerX().backTo(topBottom.back) .translateZ(thickness) val cabinet = topBottom + sides + back val slides = slideL.cut( boxDepth-slideShort ) .label( "slide" ) .alongY2().frontTo(cabinet.front+slideShort) .translateX( boxWidth - slideL.width() ).also().centerX() .topTo( thickness + 45 ) .repeatZ( drawerCount, boxHeight + gap ) return cabinet + slides } override fun build() : Shape3d { return cabinet() } }