/Electronics/BatteryTube.foocad
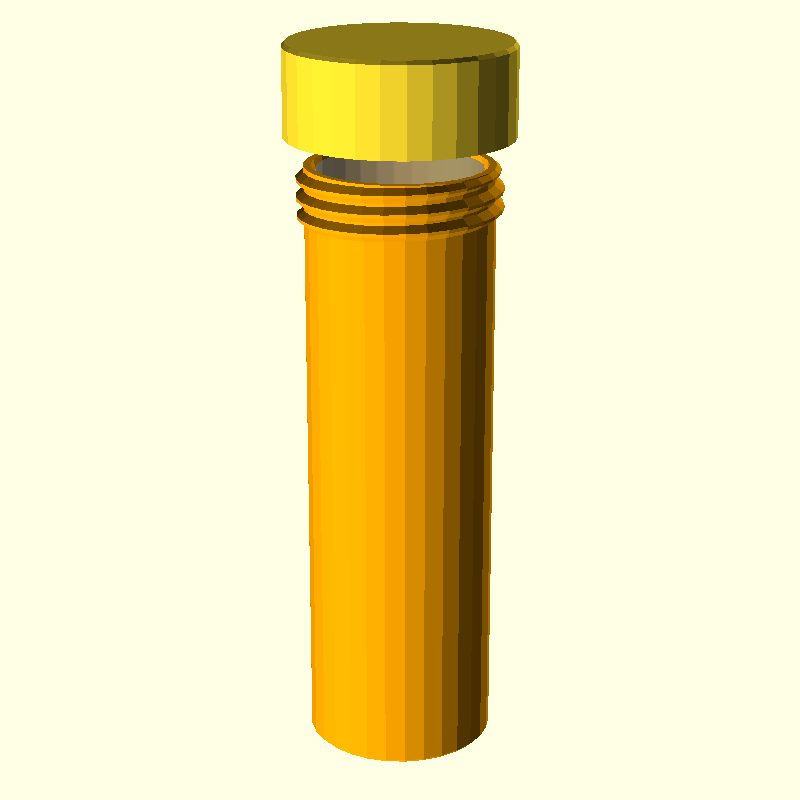
A protective case for 18650 batteries (and other sizes).
Adding machine screws
This case can also be used to electrically connect to the battery.
- Set
holeDiameter
appropriate to a machine screw - Set
extra
to account for the height of two screw head heights - Insert a screw in the body and the cap with the bolts on the outside.
- Screw the cap on tightly (which makes the electrical connection to the screw).
- Connect wires between the plastic and the bolt (ideally using a spade/ring connector, but bare wires would also work).
The cap can now be used as a switch! Unscrew slightly to break the connection.
Note. PLA isn't a good choice when using this technique, because when under stress, it deforms over time, and therefore the threads will deform, and the pressure reduces (leading to a higher resistance connection).
Remember that litium battries are dangerous, and a short circuit can lead to a fire. Don't leave any bare metal exposed.
import uk.co.nickthecoder.foocad.smartextrusion.v1.* import static uk.co.nickthecoder.foocad.smartextrusion.v1.SmartExtrusion.* import uk.co.nickthecoder.foocad.threaded.v2.* import uk.co.nickthecoder.foocad.cup.v1.* import static uk.co.nickthecoder.foocad.cup.v1.Cup.* @Data class BatteryTube : Model { @Custom var size = Vector2( 18, 65 ) @Custom var thickness = 0.8 @Custom var baseThickness = 1.2 @Custom var capHeight = 10 @Custom( about="Extra z space within the tube" ) var extra = 0 @Custom( about="Optional hole in the base and cap" ) var holeDiameter = 0.0 @Custom var clearance = 0.5 meth thread() = Thread.forRod( size.x + (thickness + clearance)*2, 2 ) .rodChamfer( 1 + thickness/2 ) meth caseDiameter() = size.x + thickness * 2 + clearance meth hole() : Shape3d { return if ( holeDiameter > 0 ) { Cylinder( baseThickness * 3, holeDiameter/2 ).centerZ() } else { null } } meth threads() = thread().threadedRod( capHeight - thickness ).threadsOnly() @Piece meth body() : Shape3d { val shape = Circle( size.x / 2 + thickness + clearance ) val main = Cup( shape, size.y + baseThickness + extra, thickness ) .baseThickness( baseThickness ) .outsideBottom( Chamfer( thickness/2 ) ) val threads = threads() .topTo( main.top ) return main + threads - hole() } @Piece meth cap() : Shape3d { val thread = thread() val solid = Circle( thread.diameter/2 + thread.clearance + thickness ) .smartExtrude( capHeight ) .bottom( Chamfer( thickness/2 ) ) val inside = thread.threadedHole( capHeight - baseThickness ) .chamferStart(false) .translateZ( baseThickness ) return solid - inside - hole() } @Piece meth both() = body().rightTo(-1) + cap().leftTo(1) @Piece( print="both" ) override fun build() : Shape3d { val body = body().color( "Orange" ) val cap = cap() .rotateX( 180 ) .bottomTo( body.top + 3 ) val battery = Cylinder( 65, 18/2 ) .bottomTo( thickness ) .previewOnly() return body + cap + battery } }