/Electronics/USBBackPlate.foocad
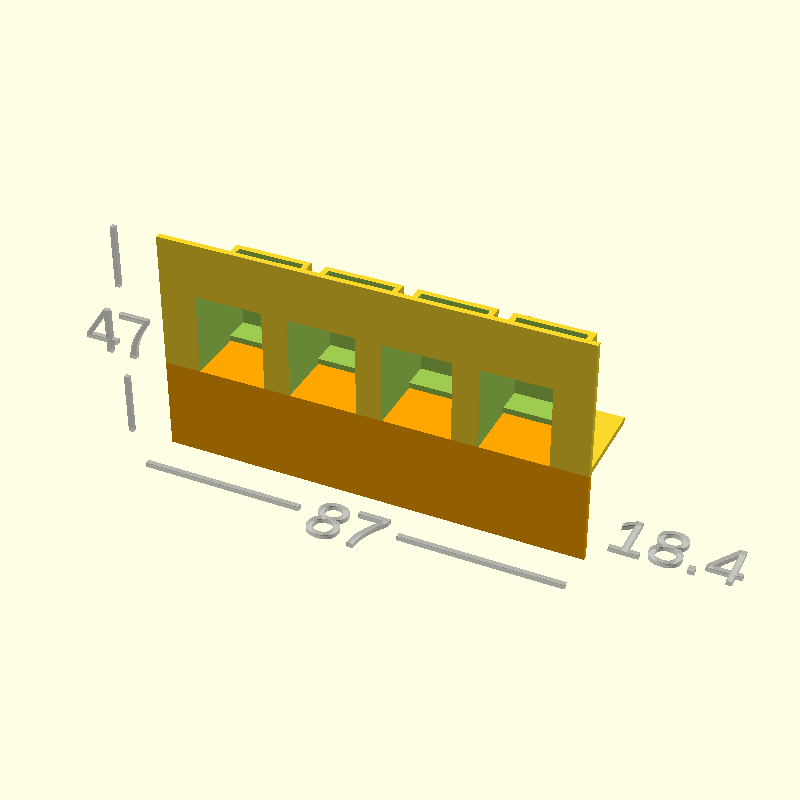
A simple housing for some double usb sockets, which are designed to be soldered onto a circuit board. However, I don't need the circuit board, and want to attach them to the back of a box.
The sockets I used are cheap on ebay : https://www.ebay.co.uk/itm/254378889580 Titled Dual USB 2.0 Type A Female Socket PCB Jack Connector SMT (despite them not being SMT components!)
I only want them for power delivery, and I'll be soldering wires directly to the pins.
The part comes in two pieces, to be glued together after the sockets are installed
Solder the wires, then rotate each socket into the boxes, adding a dab of glue to keep them in place. Avoid gluing the spingy parts!
Glue the "extra" piece to the top of the "main" piece.
import static uk.co.nickthecoder.foocad.layout.v1.Layout2d.* import static uk.co.nickthecoder.foocad.layout.v1.Layout3d.* class USBBackPlate : AbstractModel() { @Custom( about = "The size of the USB socket (the default is the size of a double socket I found on ebay" ) var socketSize = Vector3( 13.9,17.2,15.6 ) @Custom( about="The number of (double) sockets") var socketCount = 4 // Ooops the gap is between the BOXES, not the sockets, so if you change the thickness, // you need to change the gap by twice as much to keep the same overall size. Sorry. @Custom( about="The distance from one socket to its neighbour" ) var gap = 3 @Custom( about="The thickness of all of the parts" ) var thickness = 1.2 @Custom( about="The size of all panel" ) var panelWidth = 87 @Custom( about="Margin below the sockets" ) var panelBottom = 19 @Custom( about="Margin above the sockets" ) var panelTop = 10 fun socket() : Shape3d { val main = Cube( socketSize ).centerX() val holes = Cube( 10,6,4 ).centerX().frontTo(main.front - 1) .topTo( main.top - 3 ) .translateZ(-6).also() return (main - holes).previewOnly() } @Piece fun box() : Shape3d { val box = Cube( socketSize + Vector3( thickness, thickness/2, thickness ) *2 ) .centerX().frontTo(0) val middle = Cube( socketSize ) .centerX().frontTo(-0.01) .bottomTo(thickness) val noBottom = Cube( socketSize.x, socketSize.y - 3, socketSize.z ) .centerX() .backTo(middle.back) .bottomTo(-1) val noTop = Cube( socketSize.x, 12, thickness + 2 ) .centerX() .frontTo( -0.1 ) .topTo( box.top + 0.01) val one = box - middle - noBottom - noTop return one } @Piece fun main() : Shape3d { val box : Shape3d = box().mirrorZ().bottomTo(0) val front = Cube( gap, thickness, box.size.z ).leftTo(box.right).mirrorX().also() val top = Cube( front.size.x, thickness, panelTop ).centerX().bottomTo(front.top) val all = (box + front + top).tileX( socketCount, -gap ) val leftRight = Cube( ( panelWidth - all.size.x )/2, thickness, top.top ) .leftTo( all.right ).rightTo(all.left).also() val base = Cube( panelWidth - all.size.x , socketSize.y+thickness, thickness ) .leftTo(leftRight.left).rightTo(leftRight.right).also() val base2 = Cube( gap, socketSize.y + thickness, thickness ) .repeatX( socketCount - 1, socketSize.x + thickness*2 + gap ) .leftTo( socketSize.x / 2 + thickness ) return all + leftRight + base + base2 } @Piece fun extra() : Shape3d { val main : Shape3d = main() val extra = Cube( panelWidth, thickness, panelBottom ).centerX() + Cube( (socketSize.x + gap + thickness*2) * socketCount - gap, main.size.y, thickness ).centerX() return extra.leftTo( main.left ) } @Piece fun both() : Shape3d { val main : Shape3d = main() val extra : Shape3d = extra().frontTo(main.back + 2) return main + extra } @Piece( printable = false ) override fun build() : Shape3d { return main() + extra().mirrorZ().color("Orange") } }