/FooCAD/NutsAndBolts.foocad
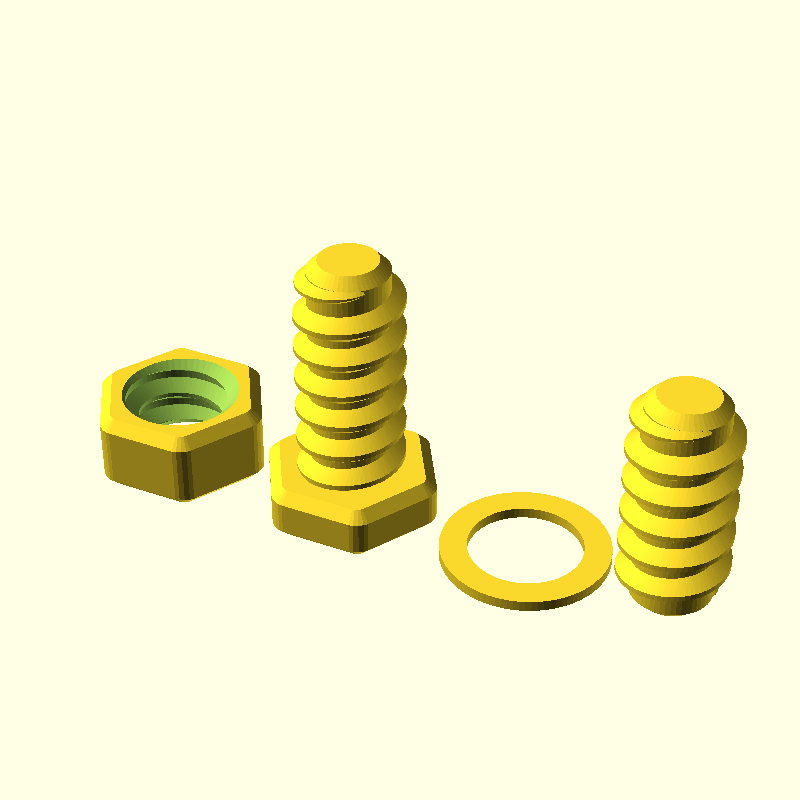
Creates nuts, bolts, washers and threaded rods using the 'standard' thread sizes.
import static uk.co.nickthecoder.foocad.arrange.v1.Arrange.* import uk.co.nickthecoder.foocad.threaded.v2.* import static uk.co.nickthecoder.foocad.threaded.v2.Thread.* class NutsAndBolts : Model { @Custom var diameter : double = 14 @Custom( about="For piece 'rod'" ) var rodLength = 30 @Custom( about="For piece 'bolt'" ) var shaftLength = 30 @Custom( about="For piece 'bolt'" ) var bareLength = 0 @Custom var headSize = Vector2.ZERO @Custom var nutHeight = 0.0 fun thread() : Thread { var thread = standardThread( diameter ) if (headSize != Vector2.ZERO) { thread = thread.headSize( headSize ) } return thread } @Custom var washerDiameter = 0.0 @Piece fun nut() = thread().nut() @Piece fun bolt() = thread().bolt( shaftLength, bareLength ) @Piece fun washer() : Washer { var washer = thread().washer() if (washerDiameter != 0.0) { washer = washer.diameter( washerDiameter ) } return washer } @Piece fun rod() = thread().threadedRod( rodLength ) @Piece override fun build() : Shape3d { return arrangeX( 2, nut(), bolt(), washer(), rod() ) } }