/Furniture/DoorStop.foocad
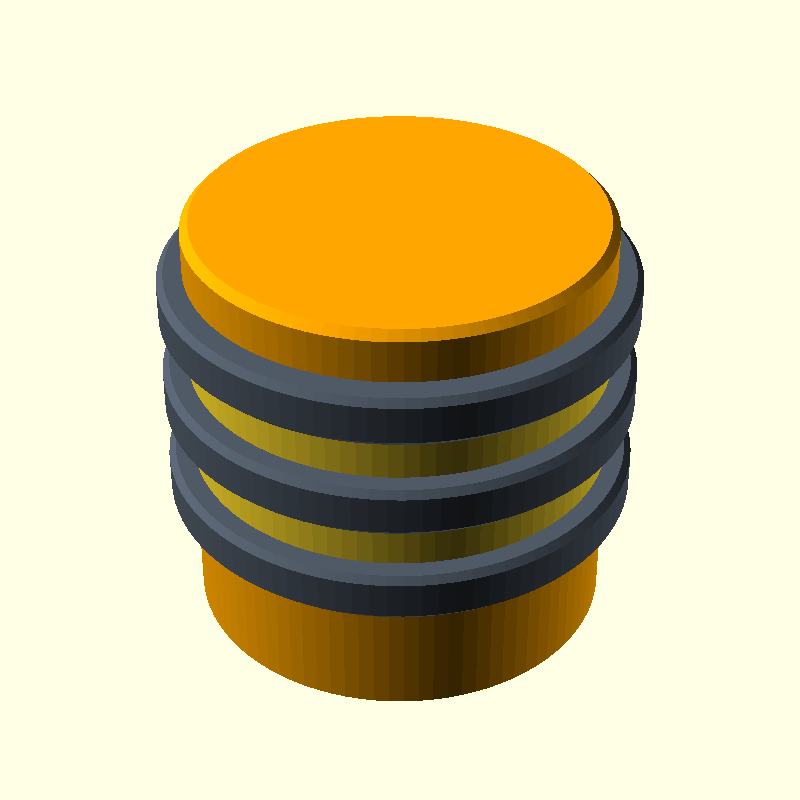
A door stop which is screwed to the floor. Made in parts, with TPU for the rubbery rings. Add a threaded rod (see rodHoleD) through the middle to screw it all together.
Assembly: Screw the bottom piece to the floor. Screw the thread rod onto the bottom piece. Stack the rings, rubber pieces and the middle section if the correct order. Finally screw on the top, which will sandwich all the parts together.
Print Notes
As it might get whacked by the door, don't be cheap on the infill nor perimeters. I used 4 perimeters, and 40% infill. For the tpu parts, I reduced this to 3 perimeters and 20% infill.
import static uk.co.nickthecoder.foocad.chamferedextrude.v1.ChamferedExtrude.* import static uk.co.nickthecoder.foocad.layout.v1.Layout2d.* import static uk.co.nickthecoder.foocad.layout.v1.Layout3d.* class DoorStop : Model { var diameter = 50 var bottomHeight = 15 var middleHeight = 6 var topHeight = 6 var topChamfer = 1 var slack = 0.5 var rubberHeight = 6 var inset = 3 var protrusion = 3 var rubberChamfer = 0.8 var rodHoleD = 5 var bigHoleD = 12 var explode = 0 @Piece fun bottom() : Shape3d { val outside = Cylinder( bottomHeight, diameter/2 ) val inside = Cylinder( rubberHeight + middleHeight/2, diameter/2 - inset - slack ) val hole = Cylinder( 10 , 3 ).bottomTo(-0.1) val biggerHole = Cylinder( 1000, bigHoleD/2 ).bottomTo( 6 ) val holes = (hole + biggerHole).translateX(diameter*0.25).repeatAroundZ(3) + Cylinder.hole( 100, rodHoleD / 2 ).center() return outside + inside.bottomTo(outside.top) - holes } @Piece fun middle() : Shape3d { val inside = Cylinder( middleHeight + rubberHeight - slack, diameter/2 - inset - slack ) val biggerHole = Cylinder( 1000, bigHoleD/2 ).bottomTo( -1 ) val holes = biggerHole.translateX(diameter*0.25).repeatAroundZ(3) + Cylinder.hole( 100, rodHoleD / 2 + 1).center() return (inside - holes) } @Piece fun ring() : Shape3d { val p = Circle( diameter/2 ) - Circle( diameter/2 - inset ) return p.extrude( middleHeight ) } @Piece fun top() : Shape3d { val outside = Circle( diameter/2 ).chamferedExtrude(topHeight, topChamfer, 0) val inside = Cylinder( topHeight + middleHeight/2+ rubberHeight, diameter/2 - inset - slack ) val hole = Cylinder.hole( 100, rodHoleD / 2 ).center().bottomTo(2) return outside + inside - hole } @Piece fun rubber() : Shape3d { return Circle( diameter/2 + protrusion ).chamferedExtrude( rubberHeight, rubberChamfer ) - Cylinder( 100, diameter/2 - inset ).centerZ() } @Piece fun pla() = layoutY( 2, layoutX( 2, ring(), ring() ), layoutX( 2, bottom(), top(), middle() ) ) @Piece fun tpu() = rubber().tileX(3, 2) override fun build() : Shape3d { val ring : Shape3d = ring() val middle : Shape3d = middle() val bottom : Shape3d = bottom() val rubber1 = rubber().bottomTo(bottom.top - rubberHeight - middleHeight/2 + explode) val ring1 = ring.bottomTo( rubber1.top + explode) val middle1 = middle.bottomTo(bottom.top + slack + explode * 3) val rubber2 = rubber().bottomTo( ring1.top + explode) val ring2 = ring.bottomTo( rubber2.top + explode ) val rubber3 = rubber().bottomTo( ring2.top + explode) val top : Shape3d = top().mirrorZ().bottomTo( middle1.top + slack + explode * 3) val rings = ring1 + ring2 val rubbers = (rubber1 + rubber2 + rubber3).color( "SlateGrey" ).darker() val pieces = (bottom + middle1 + top).color( "Orange" ) return pieces + rubbers + rings } }