/Games/Sphere8.foocad
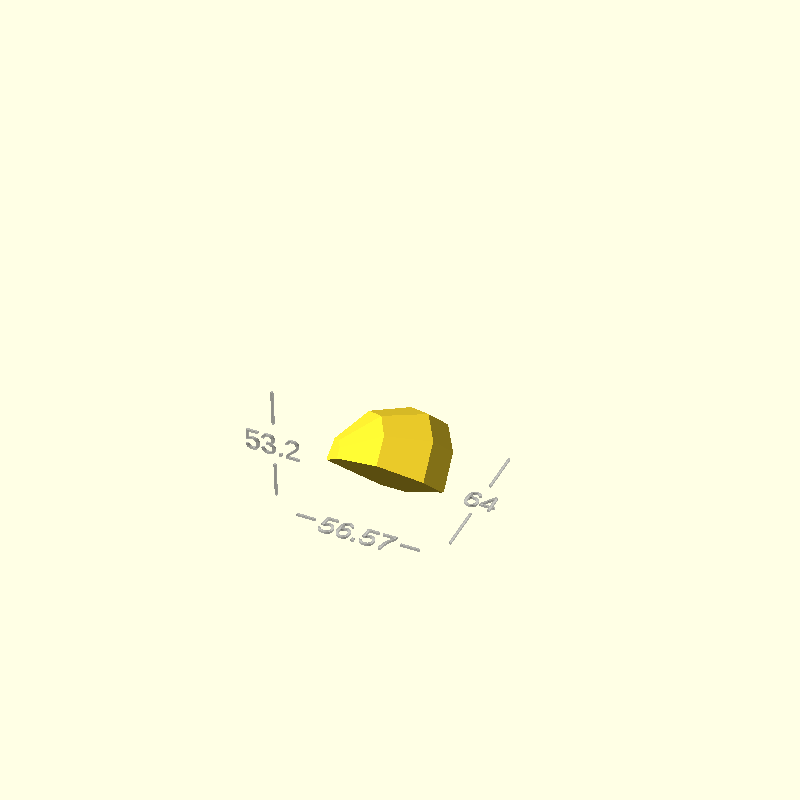
Takes a (roughly) spherical object, and cuts it into an eighth, with a flattened base, so that it can be printed wihout overhangs.
You can use this within your own models in two ways :
- extend Sphere8 and override the sphere method
- Call the static method Eighth8.eighth( shape, diameter, flatten )
import java.lang.Integer class Sphere8 : AbstractModel() { @Custom var diameter = 80 @Custom var flatten = 10 @Custom var round = false fun ball() : Shape3d { return Sphere(diameter/2).sides(12) } fun build( pieceNumber : int ) : Shape3d { return Sphere8.eighth( ball(), diameter, flatten, pieceNumber ) } @Piece fun piece0() = build(0) @Piece fun piece1() = build(1) @Piece fun piece2() = build(2) override fun build() = piece0() static fun eighth( shape : Shape3d, diameter : double, flatten : double, pieceNumber : int) = eighth( shape, diameter, flatten, pieceNumber, false ) static fun eighth( shape : Shape3d, diameter : double, flatten : double, pieceNumber : int, round : bool) : Shape3d { val angle = (pieceNumber % 4) * 90 val shape2 = if (pieceNumber >= 4) { shape.rotateX(180).rotateZ(angle) } else { shape.rotateZ(angle) } return Sphere8.eighth( shape2, diameter, flatten, round ) } static fun eighth( shape : Shape3d, diameter : double, flatten : double, round : bool) : Shape3d { val angle = Degrees.atan( Math.sqrt(2) ) val flatMask = Cube( diameter*2 ).centerXY() val result = (shape / Cube( diameter ) ) .rotateY(-45).rotateX(90-angle) .translateZ(-flatten) / flatMask val coneR = diameter*0.40 val coneH = diameter*0.29-flatten*0.5 println( "diameter = $diameter coneH = $coneH" ) val cone = ( Circle( coneR ).extrudeToPoint( coneH ).mirrorZ() + Cylinder( diameter/2, coneR ) ).translateZ(coneH-flatten*0.5) // Cube(1000).centerY().centerZ().color("Green") return result / cone } }