/Garden/BarrowHandle.foocad
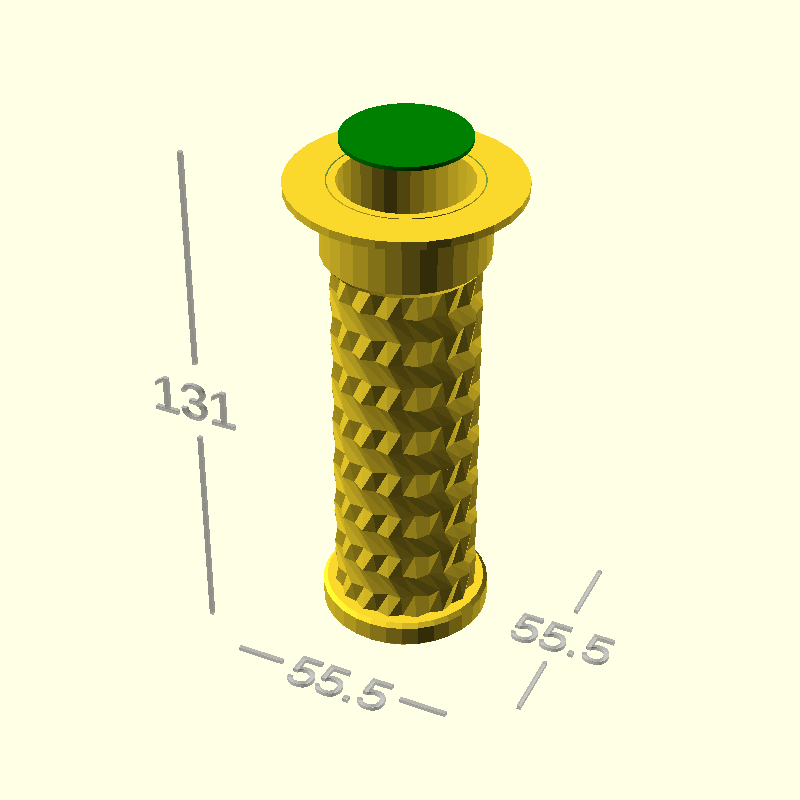
Replacement handles for my wheelbarrow.
Includes a knarlled pattern made by the union of two regular polygons rotated to form a star shape. The outside is also twisted by 10 degrees backwards and forwards.
To help printing, the handle is made in two pieces.
class BarrowHandle : AbstractModel() { var size = 31.5 // Hmm, my prints end up 1mm too small. It should be 30.5 var chamfer = 1 var length = 120 var thickness = 2 var hilt = 3 var hiltLength = 6 var stopSize = 10 var stopThickness = 2 var stopOverlap = 20 @Piece fun handle() : Shape3d { val sections = length ~/ 8 val sides = 8 val radius = size / 2 val inside = Circle.hole( radius ).sides(sides * 4) val hiltP = Circle( radius + thickness + hilt ).sides(sides * 4) val stopP = Circle( radius + thickness + stopSize ).sides(sides * 4) val stopOverlapP = Circle( radius + thickness ).sides(sides*4) val outside = Circle( radius + thickness ).sides(sides) .rotate(360/sides/2).also() .rotate(-360/sides/4) val handle = ExtrusionBuilder().apply { joinStrategy = OneToOneJoinStrategy() crossSection( hiltP.offset(-chamfer) ) forward( chamfer ) crossSection( hiltP ) forward( hiltLength-chamfer ) crossSection() forward( chamfer ) crossSection( hiltP.offset(-chamfer) ) crossSection( outside ) for (s in 1..sections) { forward( (length-hiltLength-stopOverlap-chamfer)/sections ) turnZ(if (s % 2 == 1) 10 else -10) crossSection() } crossSection( stopOverlapP ) forward( stopOverlap ) crossSection() crossSection(inside) forward( -length + thickness ) crossSection() }.build() // Cube( 100, 1, 1000 ).center() return handle } @Piece fun stop() : Shape3d { val sides = 8 val radius = size / 2 val inside = Circle.hole( radius ).sides(sides * 4) val hiltP = Circle( radius + thickness + hilt ).sides(sides * 4) val stopP = Circle( radius + thickness + stopSize ).sides(sides * 4) val stopOverlapP = Circle( radius + thickness ).sides(sides*4) val stop = ExtrusionBuilder().apply { crossSection( stopOverlapP.offset(0.3) ) forward(thickness) crossSection( stopOverlapP.offset(thickness) ) forward( stopOverlap-thickness-stopThickness ) crossSection() crossSection( stopP ) forward( stopThickness ) crossSection() }.build() - Cylinder.hole( 100, radius + thickness + 0.3 ).center() return stop } override fun build() : Shape3d { return handle() + stop().translateZ( length - stopOverlap ) + Cylinder(1,30/2).translateZ(130).color("Green") } }