/Garden/DoorClip.foocad
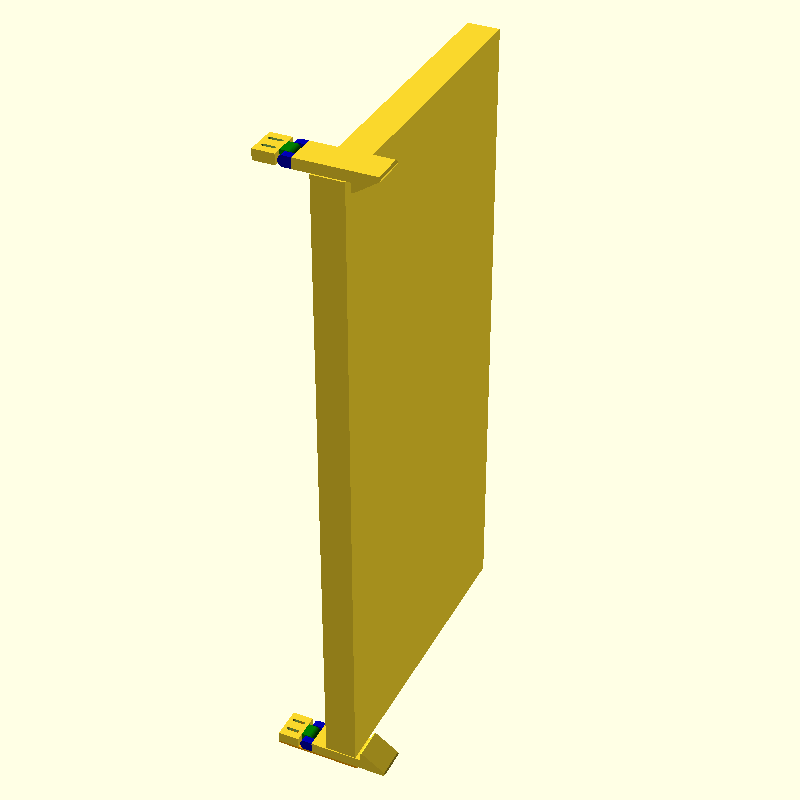
I want metal-free fixings for my door (I hate rust and paint;-) Holds a door closed.
Never finished (or printed) this.
import static uk.co.nickthecoder.foocad.extras.v1.Extras.* include Hinge.feather class DoorClip : Model { var clipSize = Vector3( 50, 30, 10 ) var triangle = Vector2( 30, 20 ) var attachmentLength = 20 var springT = 2.0 @Piece fun clip() : Shape3d { val square = Square( clipSize.x, clipSize.z ) val tri = Triangle( triangle.x, triangle.y ) .roundCorner(2,1,1) .roundCorner(1,2,2) .leftTo(square.right) val plateP = square + tri val a = Cube( attachmentLength, clipSize.y, clipSize.z ).centerY() val hinge = Hinge(clipSize.z, clipSize.z *0.5, clipSize.y, 1 ).apply { profile = Circle( clipSize.z/2 ) + Square( clipSize.z*0.7, clipSize.z ).centerY() }.bottomTo(0).leftTo(a.right) val b = plateP.extrude( clipSize.y ).rotateX(90).centerY().leftTo(hinge.right) return a - holes() + hinge + b } fun holes() : Shape3d { val hole = Cylinder( clipSize.z * 3, 1.5 ).center() val slot = hole.translateX(attachmentLength*0.6 - 2).hull( hole ).centerX() return slot .translateX( attachmentLength/2 ) .translateY( clipSize.y * 0.2 ).mirrorY().also() } /* For the clip at the bottom of the door, we use a bendy piece of plastic to hold the clip up. It only has to be springy enough to hold the weight of the clip, and be able to bend any amount downward when the clip is trodden on (to unlatch the door). */ @Piece fun spring() = Cube( clipSize.x + attachmentLength + clipSize.z + 1, clipSize.y, springT ) .centerY() - holes() override fun build() : Shape3d { val door = Cube( 30, 300, 600 ) val clip : Shape3d = clip() .rightTo(door.right+ triangle.x).frontTo(0).translateZ(-clipSize.z-1) val spring : Shape3d = spring().topTo( clip.bottom ) .leftTo( clip.left ) .frontTo(0) .color("Orange") return clip + door + clip.mirrorZ().translateZ(600) + spring } }