/Garden/JengaFeet.foocad
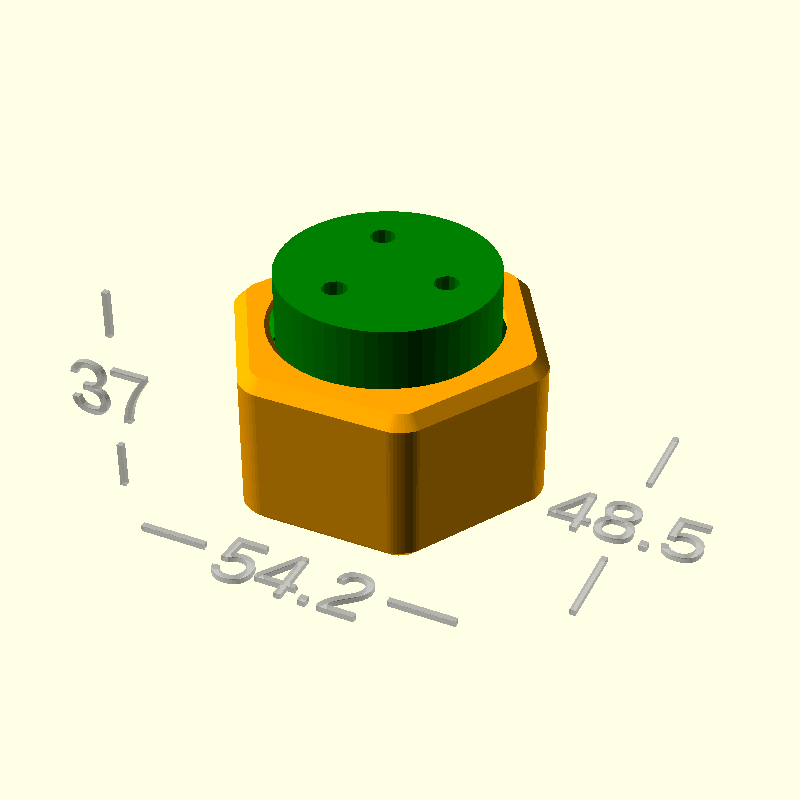
I made a giant Jenga from rounded carcassing timber from Wickes.co.uk, and was given a woodeen box, which is just about big enough to fit all of the pieces. We start the tower on top of the box (so we don't have to bend down so far, and the tower is higher), These feet fit under the box, and can be adjusted to make the platform level (or deliberately angled if required!)
See also Hardward/AdjustableFeet.foocad, which is slightly different (and uses a later version of the Threaded plugin).
import static uk.co.nickthecoder.foocad.layout.v1.Layout2d.* import static uk.co.nickthecoder.foocad.layout.v1.Layout3d.* import static uk.co.nickthecoder.foocad.chamferedextrude.v1.ChamferedExtrude.* import uk.co.nickthecoder.foocad.threaded.v2.* import uk.co.nickthecoder.foocad.screws.v3.* class JengaFeet : AbstractModel() { val diameter = 40 val pitch = 5 val height = 25 val wallThicknss = 4 val baseThickness = 2 val extraNutHeight = 0 val extraRodHeight = 10 fun threaded() = Thread( diameter, pitch ).nutHeight( height + baseThickness + extraNutHeight )//.nutChamfer( baseThickness ) // Screw is 8mm inside the "rod". // I plan on using 20mm screws, so 12mm is into the wood. fun countersink() = Countersink() .depth(height+ extraRodHeight) .recess(height + extraRodHeight - 8) @Piece fun nut() : Shape3d { val thread = threaded() val nut = thread.nut( ) val base = nut.nutShape().chamferedExtrude( baseThickness, baseThickness, 0 ) return nut + base } fun holes() : Shape3d { return countersink().mirrorZ().bottomTo(0).translateX( diameter*0.25 ) .repeatAroundZ(3) } @Piece fun rod() : Shape3d { val thread = threaded() val rod = thread.threadedRod( height ) //, 180, 30 ) val holes : Shape3d = holes() val extra = Circle(thread.coreRadius())//.sides(thread.sides) .extrude( extraRodHeight + thread.rodChamfer ) .bottomTo( height - thread.rodChamfer ) return rod + extra - holes } // A template to help mark pilot holes for the screws. @Piece fun template() : Shape3d { return (Circle( diameter/2 ) + Square( diameter/2 )).extrude(0.4) - holes().topTo(1) } @Piece( printable = false ) override fun build() : Shape3d { return nut().color("Orange") + rod().color("Green").bottomTo( baseThickness + extraNutHeight ) } }