/Garden/SHanger.foocad
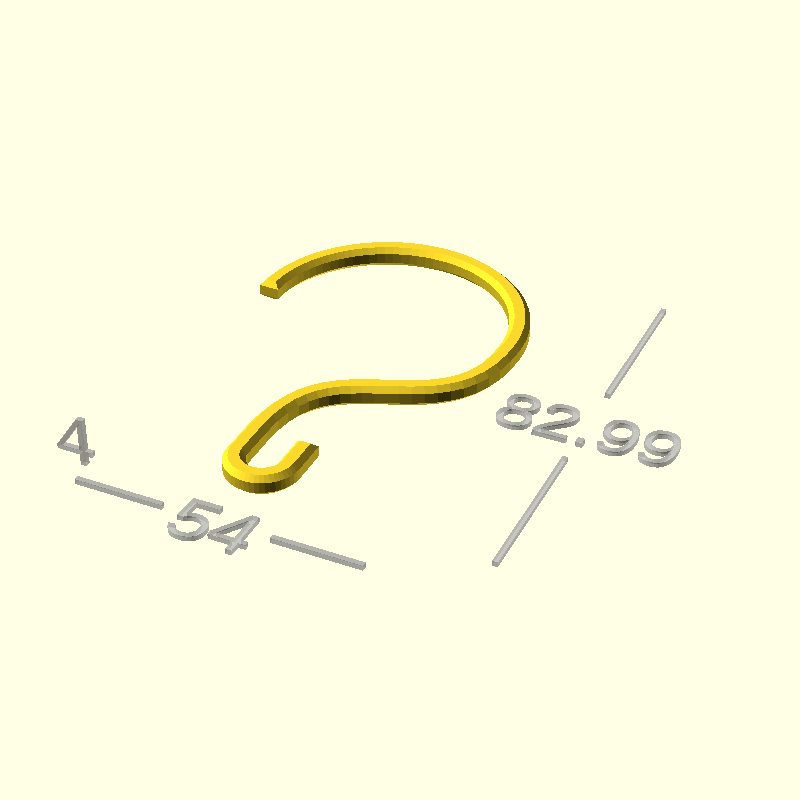
An S shaped hanger for the bird feeders.
import static uk.co.nickthecoder.foocad.pathextrude.v1.PathExtrude.* import static uk.co.nickthecoder.foocad.chamferedextrude.v1.ChamferedExtrude.* class SHanger : Model { @Custom( about="Diameter of the top (large) hook" ) var topD = 50 @Custom( about="Diameter of the bottom (small) hook" ) var bottomD = 10 @Custom( about="Extra length at the top (large) hook" ) var topExtra = 0 @Custom( about="Extra length at the bottom (small) hook" ) var bottomExtra = 7 @Custom( about="Width (x)" ) var width = 4.0 @Custom( about="Thickness (z)" ) var thickness = 4.0 @Custom var chamfer = 1.0 fun path() = PolygonBuilder().apply { moveTo(0,0) if( topExtra> 0 ) { lineBy(0,topExtra) } circularArcBy(topD, 0, topD/2, false, false) if( topExtra> 0 ) { lineBy(0,-topExtra) } bezierBy( Vector2(0,(-bottomD-topD)/2), Vector2(0,(-bottomD-topD)/2), Vector2( -topD/2-bottomD/2, -topD*0.7 - bottomD*0.7 ) ) lineBy(0,-bottomExtra) circularArcBy(bottomD, 0, bottomD/2, false, true) lineBy(0,bottomExtra) }.buildPath() override fun build() : Shape3d { return path().thickness( width ).chamferedExtrude( thickness, chamfer ) } }