/Hardware/NonZipTie.foocad
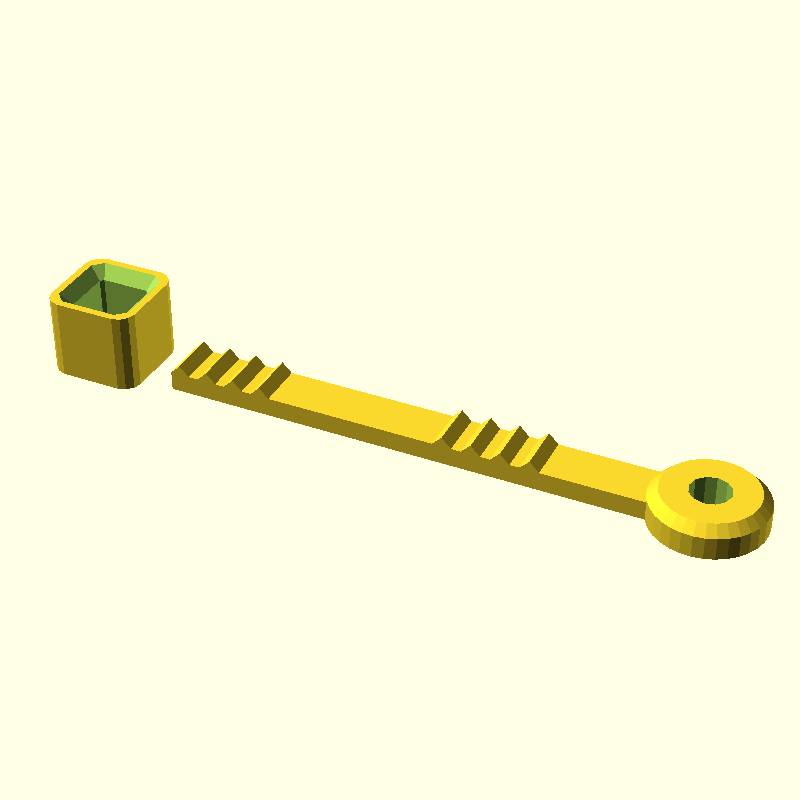
Similar use as zip ties, but can be easily removed by sliding the shroud.
Print with TPU.
import uk.co.nickthecoder.foocad.smartextrusion.v1.* import static uk.co.nickthecoder.foocad.smartextrusion.v1.SmartExtrusion.* import static uk.co.nickthecoder.foocad.layout.v1.Layout2d.* import static uk.co.nickthecoder.foocad.layout.v1.Layout3d.* import static uk.co.nickthecoder.foocad.extras.v1.Extras.* class NonZipTie : AbstractModel() { @Custom( about="Note. X is half when bent in half" ) var size = Vector3( 16, 3, 1.2 ) @Custom var toothSize = Vector2( 1.2, 0.6 ) @Custom( about="Height and thickness" ) var shroudSize = Vector2( 5, 1.2 ) @Custom var attachmentSize = Vector2( 7, 2 ) @Custom var holeDiameter = 2.5 @Custom var shroudClearance = 0.2 @Piece fun shroud() : Shape3d { val inside = Square( size.z *2 + toothSize.y, size.y ).center() .offset(shroudClearance) val outside = inside.offset( shroudSize.y ).roundAllCorners(1) return (outside - inside.roundAllCorners(shroudClearance)) .smartExtrude( shroudSize.x ).insideTop(Chamfer(shroudSize.y*0.7)) } @Piece fun strap() : Shape3d { val rounding = size.y * 0.4 val strap = Square( size.x*2, size.y ) .center() .extrude( size.z ) val attachment = (Circle( attachmentSize.x/2 ) - Circle( holeDiameter/2)) .smartExtrude( attachmentSize.y ).outsideTop( Chamfer( 0.6 ) ) .translateX( strap.right + holeDiameter/2 ) val tooth = Triangle( toothSize.x, toothSize.y, toothSize.x/2 ) .extrude( size.y ) .rotateX(90) .centerY() .bottomTo( strap.top ) val teeth = tooth .tileXWithin( shroudSize.x*1.5, toothSize.x/2 ) .rightTo( attachment.left - shroudSize.x - 1 ) .leftTo( strap.left ).also() return strap + attachment + teeth } override fun build() : Shape3d { return shroud().rightTo(-1) + strap().leftTo(1) } }