/Hardware/Suspension.foocad
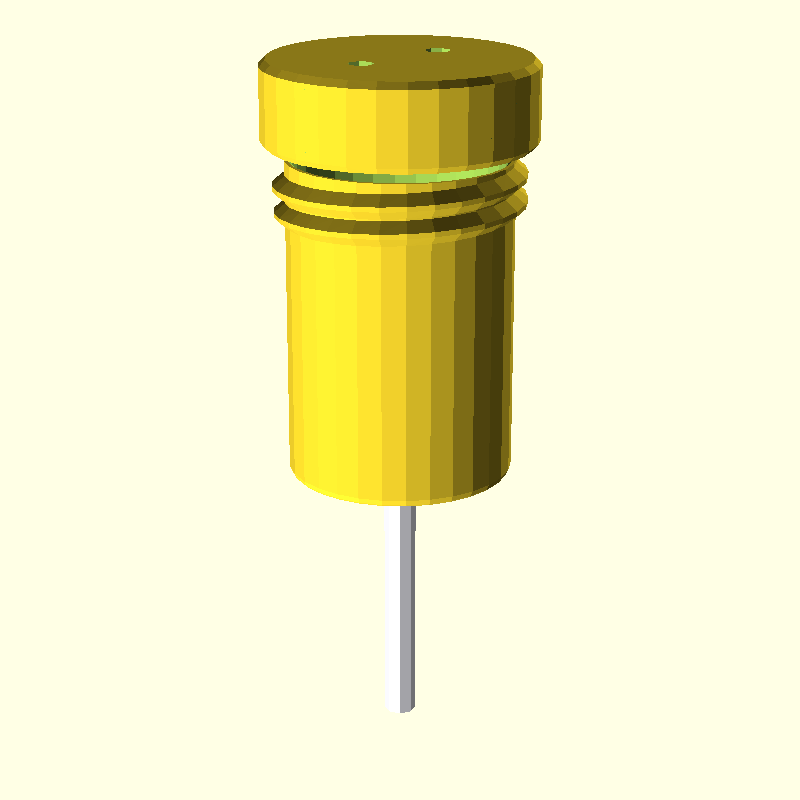
Untested
Spring suspension enclosed in a tube.
Assembly
Attach a threaded rod to piece
inner
using 2 bolts.Place the spring and piece
inner
into theouter
tube, and then attach the long end of the rod to your project, compressing the spring so that it is fully contained in the tube.Screw the cap to the other part of your project.
Bring the two parts together, and twist the tube into the cap.
import uk.co.nickthecoder.foocad.threaded.v2.* import uk.co.nickthecoder.foocad.smartextrusion.v1.* import static uk.co.nickthecoder.foocad.smartextrusion.v1.SmartExtrusion.* import uk.co.nickthecoder.foocad.cup.v1.* import static uk.co.nickthecoder.foocad.cup.v1.Cup.* class Suspension : Model { @Custom( about="Internal diameter and height" ) var size = Vector2( 30, 50 ) @Custom var thickness = 1.2 @Custom var baseThickness = 3.0 @Custom var innerHeight = 8.0 @Custom var clearance = 0.2 @Custom var threadHeight = 12.0 @Custom var stopHeight = 6.0 @Custom var rodDiameter = 5.0 meth shape() = Circle( size.x/2 + thickness ) meth thread() = Thread( size.x + thickness*4 + clearance + 4, 4 ) .rodChamfer( 1 ) @Piece meth outer() : Shape3d { val shape = shape().offset( thickness + clearance ) val hole = Cylinder( thickness + 2, rodDiameter/2 + 0.5 ).bottomTo(-1) val main = Cup( shape, size.y, thickness ) .insideTop( Fillet( thickness/2 ) ) .outsideBottom( roundedChamfer( thickness ) ) .insideBottom( Fillet( thickness * 0.75 ) ) val threaded = thread().threadedRod( threadHeight ) .threadsOnly() .topTo( main.top ) return main - hole + threaded } @Piece meth inner() : Shape3d { val shape = shape() val hole = Cylinder( baseThickness + 2, rodDiameter/2 + 0.5 ).bottomTo(-1) val main = Cup( shape, innerHeight, thickness ) .baseThickness( baseThickness ) .outsideBottom( Chamfer(0.5) ) .insideBottom( Fillet( thickness ) ) .outsideTop( Fillet( thickness * 0.75 ) ) return main - hole } @Piece meth cap() : Shape3d { val thread = thread() val threadedHole = thread.threadedHole( threadHeight ) .bottomTo( thickness ) val cap = Circle( thread.diameter / 2 + thickness + 0.5 ) .smartExtrude( threadHeight + thickness ) .outsideBottom( Chamfer(1) ) .outsideTop( Chamfer(1) ) val stopShape = shape() - shape().offset(-thickness) val stop = stopShape.extrude( stopHeight + thickness ) val screwHoles = Cylinder( thickness * 3, 2 ) .centerZ() .translateX( stopShape.left / 2 ) .mirrorX().also() return cap - threadedHole - screwHoles + stop } @Piece meth springAssembly() : Shape3d { val outer = outer() val cap = cap() .rotateX(180) .bottomTo( outer.top + 2 ) //.topTo( outer.top + thickness ) val inner = inner() .mirrorZ() .bottomTo( outer.middle.z ) //.topTo( cap.top - thickness - stopHeight ) val rod = Cylinder( outer.top + 32 , rodDiameter/2 ) .topTo( inner.top + stopHeight ) .color( "GhostWhite" ) return outer + inner + cap + rod } override meth build() = springAssembly() }