/Printer/DustFilter.foocad
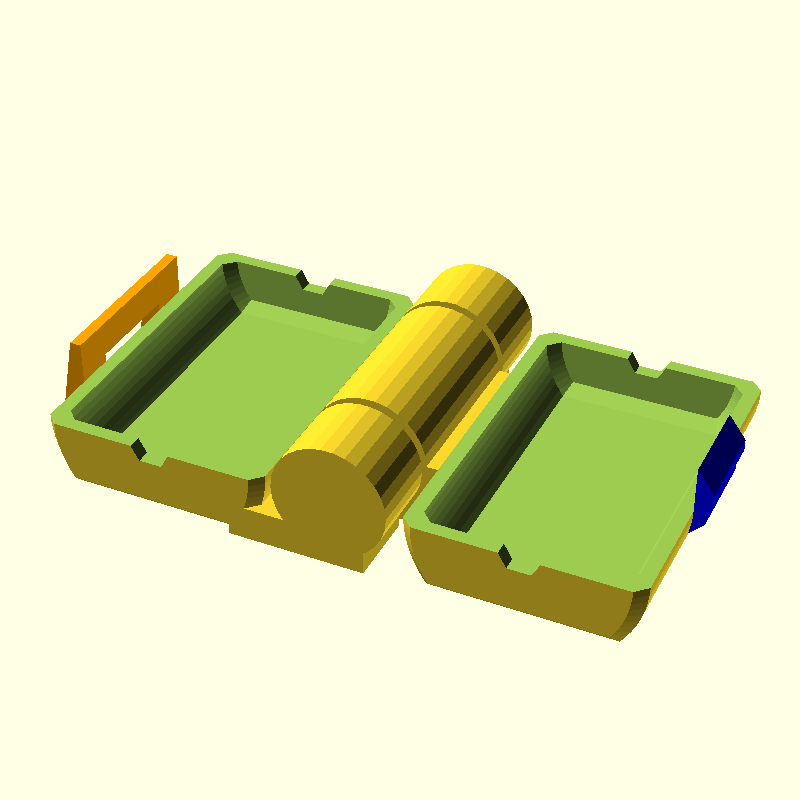
Clip this to filament just before it enters the printer to prevent dust being drawn into the hot end, where it may cause blockages, or mar the print.
I'm not 100% happy with the clip, the hinge works fine.
At some point I should probably make some reusable extensions for common tasks such as hinges and clips! At which point, this should be reworked to use them.
NOTE. On my Genius printer, this would fowl the extrusion gear, so you should also print the GeniusFilamanetGuide.
import static uk.co.nickthecoder.foocad.chamferedextrude.v1.ChamferedExtrude.* /** Clip this to filament just before it enters the printer to prevent dust being drawn into the hot end, where it may cause blockages, or mar the print. I'm not 100% happy with the clip, the hinge works fine. At some point I should probably make some reusable extensions for common tasks such as hinges and clips! At which point, this should be reworked to use them. NOTE. On my Genius printer, this would fowl the extrusion gear, so you should also print the GeniusFilamanetGuide. */ class DustFilter : Model { var holeD = 4 @Custom var width = 20 @Custom var height = 30 @Custom var thickness = 1.2 // Used to be 0.8 var claspThickness = 1.8 var slack = 0.8 fun halfHeight() = width * 0.6 fun hinge() : Shape3d { val sides = 30 val r = halfHeight()/2 val middle = Cylinder( height/2, r-1 ).sides(sides).center() + Cylinder( height - thickness*2 - slack*2, r*0.4 ).sides(sides).center() + Cube( r * 2, r, height/2 ).mirrorY().translateX(-r/2).centerZ() val end = ( Cylinder( height/4 - slack, r-1 ).sides(sides) - Cylinder( height/4-thickness, r*0.4 + slack).sides(sides).translateZ(-slack) + Cube( r * 2, r/2, height/4 -slack).mirrorX() .translateX(r/2) .translateY(-halfHeight()/2) ) .translateZ(height/4+slack) return (middle + end.mirrorZ().also()).rotateX(90).toOriginZ() // Cube( 1, 100, 100 ).center() } fun hookAndBarb() : Shape3d { val hook = PolygonBuilder().apply { moveTo(-1,-1) lineTo(1,1) lineTo(1,2) lineTo(1.5,3) lineTo(1.5,4) lineTo(0,6) lineTo(0,2) //lineTo(0,5) }.build().extrude( 8 ) .rotateX(90) .centerY() .translateX(width+halfHeight()/2) .translateZ(halfHeight()/2-2) val h = halfHeight()/2+3 val barb1 = PolygonBuilder().apply { radius(0.5) moveTo(-4,0) lineTo(2.5,0) // This is a week point lineTo(2.5,1) radius(0.0) lineTo(1,h) lineTo(0,h) radius(0.5) lineTo(0,1) radius(0) lineTo(-4,1) }.build().extrude( 16 ) .rotateX(90) .centerY() val barb = (barb1 - Cube( 6,9,4 ).centerXY().translateZ(2)) .translateX(width+halfHeight()/2+0.6) return hook.color("Blue") + barb.color("Orange").mirrorX() } fun profile( width : double ) : Shape2d { return Circle( width / 2 ) / Square( width*1.1, width*0.6 ).center() } override fun build() : Shape3d { val half = ( profile( width ).chamferedExtrude( height, 1 ).center().rotateX(90) - Cube( width*2, height*1.1, width ).centerXY() ).toOriginZ() val minus = ( profile( width-thickness*2 ).chamferedExtrude( height - thickness*2, 1 ).center() + Cylinder( height*1.2, holeD/2 ).sides(6).center() ).rotateX(90).toOriginZ().translateZ(thickness) val result = half.translateX( width/2+halfHeight()/2 ).mirrorX().also() + hinge() - minus.translateX( width/2+halfHeight()/2 ).mirrorX().also() + hookAndBarb() return result // Cube( 1, 100, 100 ).center() } }