/Tools/Clamp.foocad
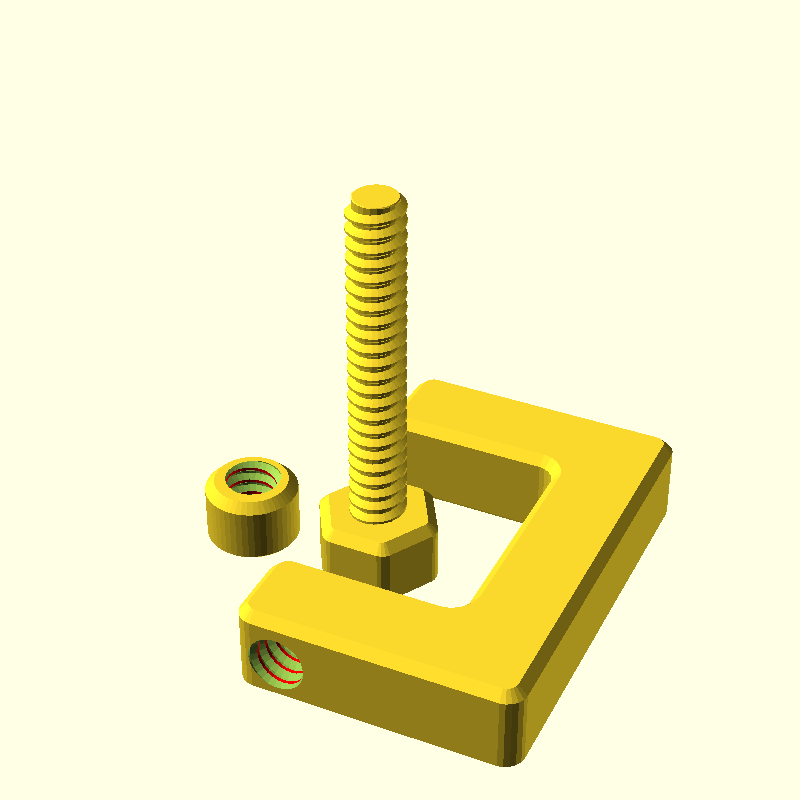
A mini clamp, useful for adding pressure when glueing small items.
The pieces are easy to print. No supports needed.
However, I recomment a brim when printing the long thin bolt, to ensure it does get knocked over.
It produces a reasonable pressure, but I'm sure it's very easy to strip the threads if you tighten a lot.
If the threads do strip, you could melt in a threaded insert, and use a regular metal bolt ;-))
import static uk.co.nickthecoder.foocad.chamferedextrude.v1.ChamferedExtrude.* import uk.co.nickthecoder.foocad.threaded.v2.* import static uk.co.nickthecoder.foocad.threaded.v2.Thread.* class Clamp : AbstractModel() { var size = Vector2(20,25) var thickness = 10 var thread = Thread(6) .headSize(Vector2(12,8)) .headChamfer(1) .rodChamfer(0.1) .clearance(0.3) @Piece fun clamp() : Shape3d { val profile = Square( size.x + thickness, size.y + thickness * 2 ).centerY() - Square( size ).centerY() val c = profile.toPolygon().roundAllCorners(2).chamferedExtrude( thickness, 1 ) val hole = thread.threadedHole( thickness*2 + 2 ) .center() .rotateX(90) .translate( thickness/2, 0, thickness/2 ) .frontTo( profile.front - 1 ) return c - hole } @Piece fun foot() : Shape3d { val foot = Circle( thickness/2 ) .chamferedExtrude( thickness*0.7, 0.4, 1 ) val hole = thread.threadedHole( thickness ).clipped().bottomTo( 1 ) return foot - hole } @Piece fun bigFoot() : Shape3d { val foot = Circle( (size.x+thickness)/2-1 ).center().roundAllCorners(1) .chamferedExtrude( thickness*0.7, 0.4, 1 ) val hole = thread.threadedHole( thickness ).clipped().bottomTo( 1 ) return foot - hole } @Piece fun bigBase() : Shape3d { val base = Square( size.x+thickness-2 ).center().roundAllCorners(1) .chamferedExtrude( thickness*0.7, 0.4, 1 ) val slot = Cube(100, thickness+0.3, 100).centerY() .leftTo(base.left + thickness) .bottomTo(2) return base - slot } @Piece fun bolt() : Shape3d { return thread.bolt( size.y + thickness + 2 ) } @Piece fun withBigFoot() : Shape3d { val big = bigFoot().frontTo(1) + bigBase().backTo(-1) return big.translateX( size.x*2 ) + bolt() + clamp().translateX(-thickness/2) } override fun build() : Shape3d { return foot().translateX(-thickness*1.5) + bolt() + clamp().translateX(-thickness/2) } }