/Tools/HoleSizes.foocad
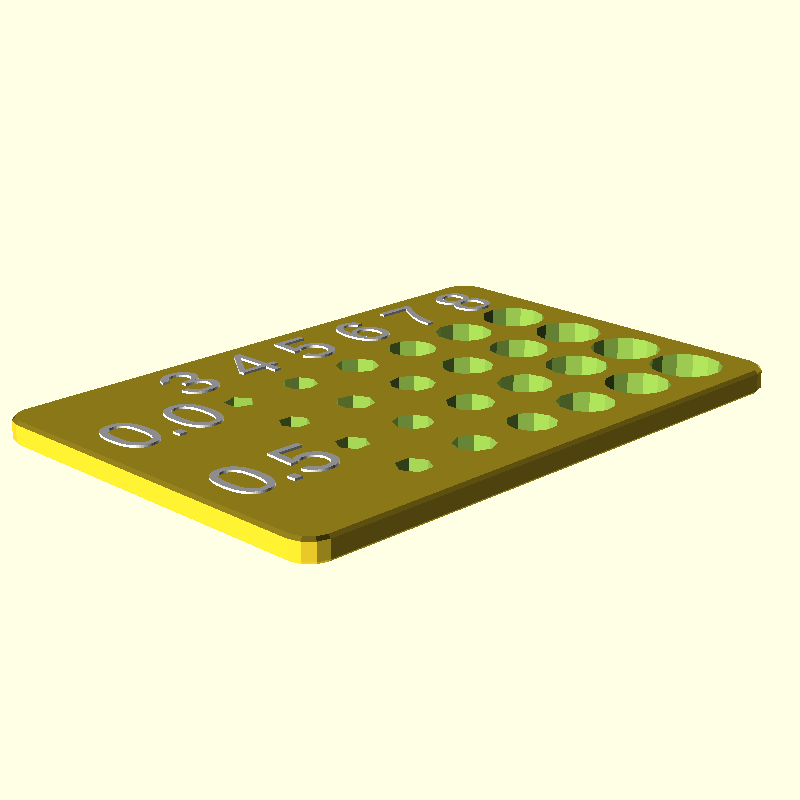
Holes in various sizes.
I use this to choose the correct size hole for a piece of hardware. e.g. If I have an M4 bolt, I can place it in various holes to find the fit I want.
I've included an upright version too, because the tollerances are different in the Z axis. This version uses Circle.safeOverhang().
M4 Bolts
My first use-case was the best hole-size for an M4 bolt to self-tap into. Vertical holes are tight with a 4.0mm. Horizontal holes are a tad too loose with a 4.0mm hole, 3.75mm was better (but tight). Printed with a 0.6mm nozzle using my "fast" settings (0.4mm layer height).
Important
All holes are defined using Circle.hole(radius), which will be LARGER than just Circle( radius ).
If you print horizontal holes, beware that use of Circle.safeOverhang() will also make a difference to the size of the holes. Without it, filament may droop, leading to a smaller hole. For small diameters, this isn't an issue.
import uk.co.nickthecoder.foocad.smartextrusion.v1.* import static uk.co.nickthecoder.foocad.smartextrusion.v1.SmartExtrusion.* import uk.co.nickthecoder.foocad.extras.v1.* import static uk.co.nickthecoder.foocad.extras.v1.Extras.* class HoleSizes : Model { @Custom var height = 3 meth chart(upright : bool) : Shape3d { var holes : Shape2d = Union2d() var text : Shape2d = Union2d() val spacing = 9.5 for ( size in 3 .. 8 ) { text += Text( "$size", 8 ) .centerXTo( size * spacing ) .frontTo( 3 ) .toPolygon() for ( adjustment in 0 .. 3 ) { val diameter = size + adjustment/4 val hole = if (upright) { Circle.hole( diameter /2 ).safeOverhang() } else { Circle.hole( diameter /2 ) } .centerXTo( size * spacing ) .centerYTo( -adjustment * spacing ) holes += hole } } for ( adjustment in 0 .. 2 step 2) { text += Text( "${adjustment/4}", 8 ) .centerYTo( -adjustment * spacing ) .rightTo( holes.left -2 ) } val rectangle = (holes+text).boundingSquare().offset( 3 ) .roundAllCorners(3) return (rectangle - holes).smartExtrude( height ).outside(Chamfer(0.4)) + text.extrude( 0.3 ).bottomTo( height ).color("White") } @Piece @Slice( brimWidth = 10 ) meth upright() : Shape3d { return chart( true ).rotateX(90).bottomTo(0) } override fun build() : Shape3d { return chart( false ) } }