/Tools/PunchHandle.foocad
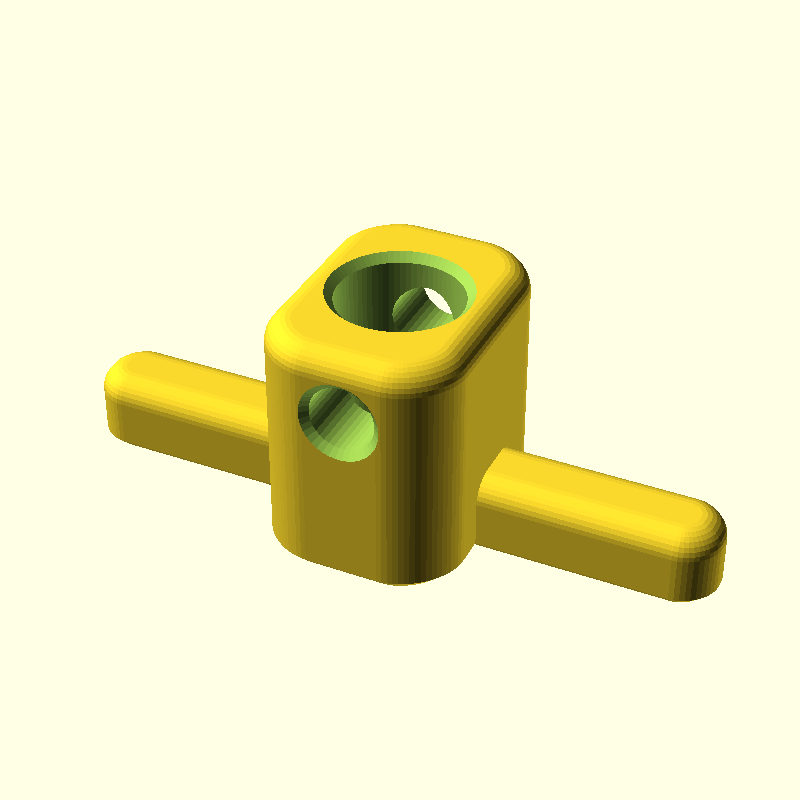
I have a punch to make holes in fabric for eyelets. Rather than hit it with a hammer, I wonder if I can use it like a drill bit. The 12mm punch is too big for my cordless drill, so maybe a simple handle is good enough???
The side has a hole for an M6 threaded insert, for a bolt to work like a grub screw, holding the punch in place.
Melt the threaded insert while the punch is in-place. Otherwise, the melted plastic may stray into the hole for the punch.
import static uk.co.nickthecoder.foocad.chamferedextrude.v1.ChamferedExtrude.* import static uk.co.nickthecoder.foocad.chamferedextrude.v1.ChamferedExtrude.* import static uk.co.nickthecoder.foocad.layout.v1.Layout2d.* import static uk.co.nickthecoder.foocad.layout.v1.Layout3d.* import uk.co.nickthecoder.foocad.smartextrusion.v1.SmartExtrusion import static uk.co.nickthecoder.foocad.smartextrusion.v1.SmartExtrusion.* import static uk.co.nickthecoder.foocad.extras.v1.Extras.* class PunchHandle : Model { @Custom( about="Diameter of the tool, with extra for clearance (0.2mm?)" ) var diameter = 15.2 @Custom var thickness = 1.2 @Custom var handleSize = Vector3( 80, 10, 10 ) @Custom var middleSize = Vector3( 24, 32, 30 ) @Custom var grubScrewDiameter = 8 @Piece fun cap() : Shape3d { val outerRadius = diameter/2 + 1 val innerRadius = diameter/2 val tube = Cylinder( 10, outerRadius ) - Cylinder( 10, innerRadius ).translateZ(1) val small = ( Sector( outerRadius,-20,20 ) - Circle( innerRadius ) ) .smartExtrude(6) .scaleTop( Vector2(0.8, 1) ) .topTo(0).mirrorZ().also() .bottomTo( tube.top ) val big = ( Sector( outerRadius, 25, 360-25 ) - Circle( innerRadius ) ) .smartExtrude(12) .bottomTo( tube.top ) return tube + big + small } @Piece fun cap2() : Shape3d { val outerCircle = Circle( diameter/2 + 1 ) val innerCircle = Circle( diameter/2 ) val thinScale = 0.97 val fatScale = 1.05 return ExtrusionBuilder().apply { crossSection( outerCircle.scale(fatScale) ) forward( 8 ) crossSection() forward( 8 ) crossSection( outerCircle.scale( fatScale, thinScale ) ) forward( 8 ) crossSection( outerCircle.scale( thinScale, fatScale ) ) crossSection( innerCircle.scale( thinScale, fatScale ) ) forward( -8 ) crossSection( innerCircle.scale( fatScale, thinScale ) ) forward( -8 ) crossSection( innerCircle.scale(fatScale) ) forward( -7 ) crossSection( innerCircle.scale(fatScale) ) }.build() } override fun build() : Shape3d { val fillet = handleSize.y * 0.3 val handleProfile = Square( handleSize.x, handleSize.y ) .center() .roundAllCorners( handleSize.y / 2-0.5 ) val middleRounding = Math.min( middleSize.y - diameter -1, middleSize.x - grubScrewDiameter - 1 )/2 val middleProfile = Square( middleSize.x, middleSize.y ) .center() .roundAllCorners( middleRounding ) val hole = Circle( diameter/2 ) .chamferedExtrude( middleSize.z -1, 0, -1 ) .bottomTo( 1.01 ) val grubScrewHole = Circle( grubScrewDiameter/2 ) .chamferedExtrude( middleProfile.size.y + 0.2, -1 ) .rotateX(90) .centerY() .topTo( middleSize.z - fillet ) val handle = handleProfile .smartExtrude( handleSize.z ) .chamferBottom(1.4) .smartFilletTop( fillet ) val middle = middleProfile .smartExtrude( middleSize.z ) .chamferBottom(1.4) .smartFilletTop( fillet ) // Allow the piece to be printed with sparse infill, // and still be strong // (The slicer will add extra perimeters and solid layers near these tiny voids) val forceExtra = Cylinder( hole.bottom - 0.8, 0.1 ) .sides(4) .bottomTo( 0.4 ).color("Red") val forceExtras = forceExtra + forceExtra .translateX( diameter * 0.45 ) .repeatAroundZ( 12 ) + forceExtra .translateX( diameter * 0.15 ) .repeatAroundZ( 6 ) return handle + middle - hole - grubScrewHole - forceExtras } }