/Tools/ScrewSizes.foocad
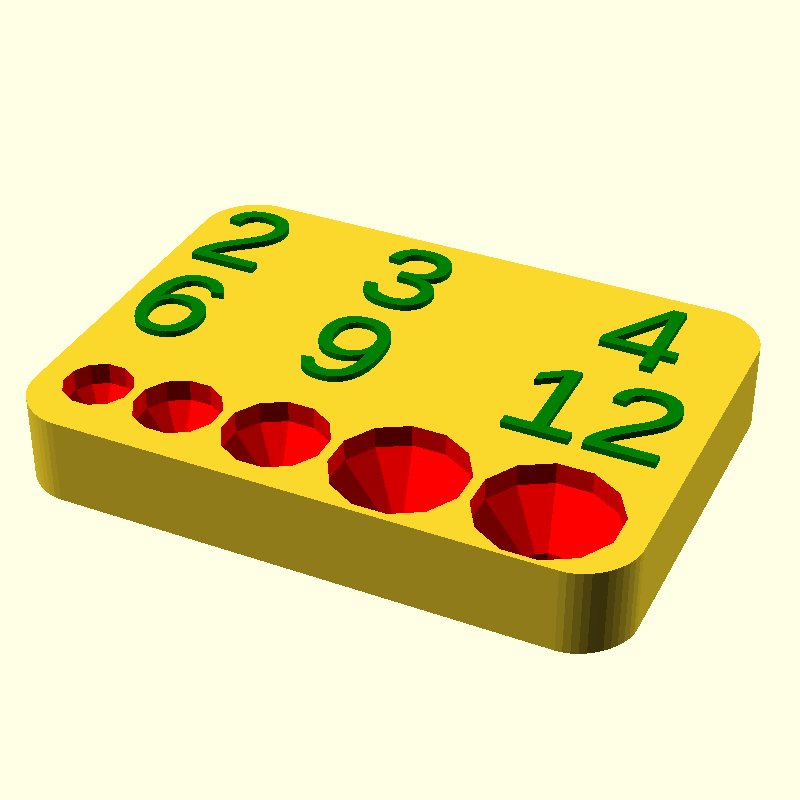
Screw Size Guage
For use with FooCAD's "Screws" extension.
Helps you choose the holeDiameter and headDiameter paramaters of Countersink / KeyholeHanger for the screws you plan to use.
import static uk.co.nickthecoder.foocad.screws.v3.Screws.* import static uk.co.nickthecoder.foocad.arrange.v1.Arrange.* class ScrewSizes : Model { val blockSize = Vector3(55,40,8) fun labels( holeDiameter : int, headDiameter : int ) : Shape3d { val a = Text( "$holeDiameter" ).frontTo(-1) val b = Text ( "$headDiameter" ).backTo(2) return (a.centerX() + b.centerX()).extrude(0.6).color("green") } fun holes( holeDiameter : double, headDiameter : double ) : Shape3d { return KeyholeHanger(holeDiameter, headDiameter) .backTo(blockSize.y/2 - 5) + Countersink(holeDiameter, headDiameter) .recess(2) .depth(blockSize.z) .bottomTo(0) .translateY(-blockSize.y/2 + 2) } override fun build() : Shape3d { val a = holes( 2, 6 ) val b = holes( 2.5, 7.5 ) val c = holes( 3, 9 ) val d = holes( 3.5, 11.5 ) val e = holes( 4, 12 ) val all = arrangeX( a, b, c, d, e ).centerXY() val block = Square( blockSize.x, blockSize.y ) .roundAllCorners(5) .center() .extrude( blockSize.z ) val labels = ( labels(2, 6).centerXTo(-22) + labels(3, 9).centerXTo(-5) + labels(4, 12).centerXTo(18) ).bottomTo(block.top).translateY(6) return block - all + labels } }